Salesforce and OpenAI API integration: a step-by-step guide
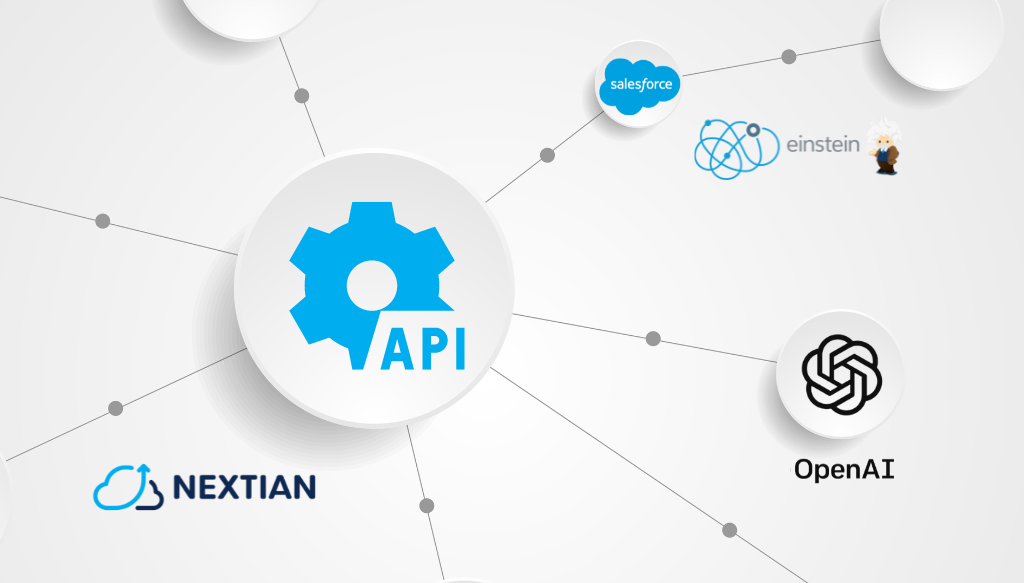
Artificial Intelligence (AI) is rapidly transforming the IT landscape with applications such as chatbots, response generation, root cause analysis, and more, promising increased productivity and streamlined automation.
With a multitude of options from vendors such as OpenAI, Google, AWS, and others, choosing the right AI solution can be overwhelming for customers.
Salesforce offers its own AI, Einstein, but many businesses might prefer OpenAI due to its significant market share — 26.30% in the Artificial Intelligence category compared to Einstein GPT’s 0.02%. OpenAI may also be better suited for specific applications due to superior models, better performance, and others. Additionally, Einstein GPT leverages OpenAI for generative AI, so a native implementation might be preferable.
This article offers a comprehensive step-by-step guide for integrating Salesforce with OpenAI’s generative API via REST. In the provided example, OpenAI will be used to help support teams in generating case summaries for customer updates.
Considerations
Before implementing the integration of Salesforce with OpenAI (or any other AI), it is essential to consider the following factors:
GPT model selection
GPT, short for Generative Pre-trained Transformer, is a family of language models capable of understanding and generating natural language text.
OpenAI offers several models, including:
- GPT-3.5: An older model on which the free ChatGPT is based.
- GPT-4o mini: Optimized for performance and lower resource consumption.
- GPT-4o: Designed for complex tasks, generating higher-quality text.
When logging into ChatGPT, users can select a model (this will be part of the configuration in our example):
Different models produce different outputs; for example, GPT-4o is known for generating more “beautiful” text than GPT-3.5. Additionally, more complex models generally have longer response times. Also, not all models are available in all GPT plans. We recommend experimenting with different models, prompts and input data to obtain the best results, before coding.
Once the model has been selected, it is best to store the selection as a configuration parameter, e.g., in custom metadata. This will allow Salesforce administrators to easily change the model as needed.
Training own model
Large organizations with terabytes of historical data (e.g., hundreds of thousands of customer support cases and their histories) can use that information to train their own GPT models. Feeding proprietary information (which is usually not publicly available) will yield better responses, closely aligned with historical data, and be valuable for root-cause analysis.
API call volumes
OpenAI charges API calls by volume, so it’s important to manage costs. Apart from selecting a good plan and negotiating terms, we recommend deploying the integration with a limited user base (e.g., a smaller, dedicated team within support) and evaluating usage from there.
Data security
To ensure data ownership and prevent customer information from being used to train public GPT models (effectively disclosing it), ensure you have a plan to own and control your data. The ChatGPT Team, ChatGPT Enterprise plans, and API Platform do not train GPT with your business data. For more details, refer to OpenAI’s enterprise privacy policies.
Implementation
Once all the considerations outlined above have been addressed, we can start the implementation:
Step 1 | Purchase your OpenAI API plan and configure API access |
The API must be purchased separately from the interactive ChatGPT plan (also, there are no free API calls).
Follow these steps to set up your API:
- Log into the Open AI Platform.
- Choose API.
- Go to Settings → Organization → Billing and configure your payment methods and spending limits.
- If you prefer not to use the Default project, create a new OpenAI API project from the menu located at the top-left side of the screen.
- Once the project has been created, select Organizational overview from the same menu and choose API keys.
- Create a new secret key for the project (it should start with the sk- prefix) and copy its value. This key will be used to authenticate with REST APIs.
It is generally recommended to create a separate OpenAI project (and secret key) for each application to control usage limits and spending.
Step 2 | Validate API access |
Use a REST client to validate your OpenAI secret key.
We recommend using CURL along with the ready-to-use examples provided in the OpenAI API Reference.
Step 3 | Add OpenAI API in Salesforce Remote Site Settings |
To ensure accessibility of the OpenAI API from APEX code in Salesforce, it must be configured as a trusted site.
Navigate to Salesforce Setup, then to Remote Site Settings, and add https://api.openai.com as a trusted endpoint.
At this point, everything is ready for development. However, we recommend prototyping with ChatGPT first, as it is faster, easier, and less expensive compared to immediate coding.
Step 4 | Identify and retrieve input data |
When using AI to generate generic responses (e.g., asking ChatGPT to write a meeting thank you email), additional information is typically not needed.
However, to create accurate case summaries, we require detailed case information such as status, open date, comments, resolution, and other relevant data a support team member would reference when drafting a summary. This data, along with a prompt (instructing GPT on what to do with the data), will be sent as a request to the API to generate the response.
Case data like Case Number, Subject, Description, public comments and others can be retrieved using a SOQL query in the Salesforce Developer Console (Developer Console → Query Editor).
An example case query might look like this:
SELECT Id, Subject, Description, CreatedDate, Age_Formula__c, Status, (SELECT Id, CreatedDate, Body__c FROM Comments__r) FROM Case WHERE CaseNumber =<your case number here>;
Since OpenAI can process JSON input, the simplest way to pass information to GPT is by converting the SOQL output to JSON using Salesforce Apex. This conversion can also be done within the Salesforce Development console (Developer Console → Debug → Open Anonymous Execute Window).
The Apex code for the query above would look like this:
List<Case> cases = [SELECT Id, Subject, Description, CreatedDate, Age_Formula__c, Status, (SELECT Id, CreatedDate, Body__c FROM Comments__r) FROM Case WHERE CaseNumber =<your case number here>]; System.debug(JSON.serializePretty(cases, true).stripHtmlTags()); // This creates JSON without HTML tags
The Apex code will produce output similar to this:
{ "attributes" : { "type" : "Case", "url" : "/services/data/v61.0/sobjects/Case/500d10000013mNkAAI" }, "Id" : "500d10000013mNkAAI", "Subject" : "Generator not working!", "Description" : "After 3 months my generator stopped working!", "CreatedDate" : "2024-08-02T07:08:15.000+0000", "Age_Formula__c" : 0.26, "Status" : "New", ...
This data, along with a prompt, can then be passed directly into ChatGPT.
Important The effectiveness of generative AI is highly dependent on the quality of the input data. This principle is akin to business analytics, where the utility of reports relies on the presence, accuracy, and accessibility of data. If the data quality is lacking, the recommended approach is to first address and rectify the data issues before embarking on AI projects. |
Step 5 | Prepare prompts and test them with input data |
An interactive GPT session typically looks like this:
Start with an initial prompt → Provide additional information (if needed) → Obtain the initial output from GPT → Refine the output with secondary prompts → Use the output
Automating this process involves the same steps. Begin with an initial prompt, which might look like this:
Using the provided information, respond by adhering to the structure demonstrated in the examples given while keeping responses relatively short. Please disregard the content within the examples and substitute it with the content from the provided prompt.
Example:
Shortened high-level description
Summary of actions taken so far
Current status based on last action.
End of Example.
Information:
Once the initial prompt is entered into the ChatGPT window, GPT will ask for additional information. At this stage, JSON data obtained in Step 4: Identify and retrieve input data can be pasted into the ChatGPT window. Hit enter and you will get a result!
Experiment with selecting various models, prompts, and combinations of datasets to refine the results further.
Step 6 | Putting it all together/implementing in Salesforce |
With the GPT model, input data, and prompts selected and prepared, the implementation process in Salesforce can begin.
Below is the recommended order of implementation tasks:
Configuration Metadata. Store your API token, model selection, call timeouts as configuration items within custom metadata. This will enable easy adjustments during and after development.
Initial Prompts. Store your initial prompts within the configuration as well. This setup is sufficient for an MVP (Minimum Viable Product). However, for a production-grade solution, it is advisable to create an administrative UI allowing to update or add prompts as needed.
Data Retrieval SOQL/APEX. Develop APEX code to retrieve the necessary data using SOQL along with conversion to JSON. Depending on your needs, you can either create a global method for data retrieval or divide the logic based on different prompts. Alternatively, you can store queries for dynamic execution, though this will require additional effort.
OpenAI API Calls. Create APEX code interacting with OpenAI chat API via REST. Since the data is handled in text or JSON format, the coding involved should be relatively straightforward.
Create the UI. Develop a Lightning action with a corresponding user interface (UI) to achieve the following:
- Initial Prompt Selection: Allow users to select an initial prompt, with the option to modify the prompt as needed.
- Initial Output Generation: Retrieve additional information and call OpenAI to generate initial output based on the prompt.
- Refinement Process: Enable users to refine the generated output using secondary prompts, which can be selected from templates or provided ad-hoc by the user.
- Output Handling: Provide actions for managing the output such as Save as Comment, Send as Email, or other necessary actions.
- Debugging Support: Add thorough debug logs in the web browser console to assist with diagnostics & troubleshooting.
An example implementation might look like this:
In the example above, GPT model selection is available; however, it is recommended to hide this option for regular users.
Summary
Today’s businesses cannot afford to ignore AI, because it drives significant competitive advantages. Without AI, businesses risk falling behind in innovation, efficiency, and customer satisfaction, ultimately compromising their growth and profitability in an increasingly digital and data-driven world.
There is a lot of hype and many myths surrounding AI, making the decision-making process and technology adoption challenging for end customers. However, as demonstrated above, simple implementations can be quite straightforward and are not significantly different from adopting any other technology.
About Nextian
Nextian offers software products and development services helping cloud & communications providers improve their Quote-to-Cash to drive revenue growth and customer base retention.
Our offerings include advanced AI solutions in the areas of customer support (generative AI, ticket routing), and analytics (sentiment scoring, churn prediction, skills-based matching), especially in conjunction with CRMs and customer support systems.
Contact us today to find out how we can help your business grow!