Dynamic calling of static APEX methods in Salesforce
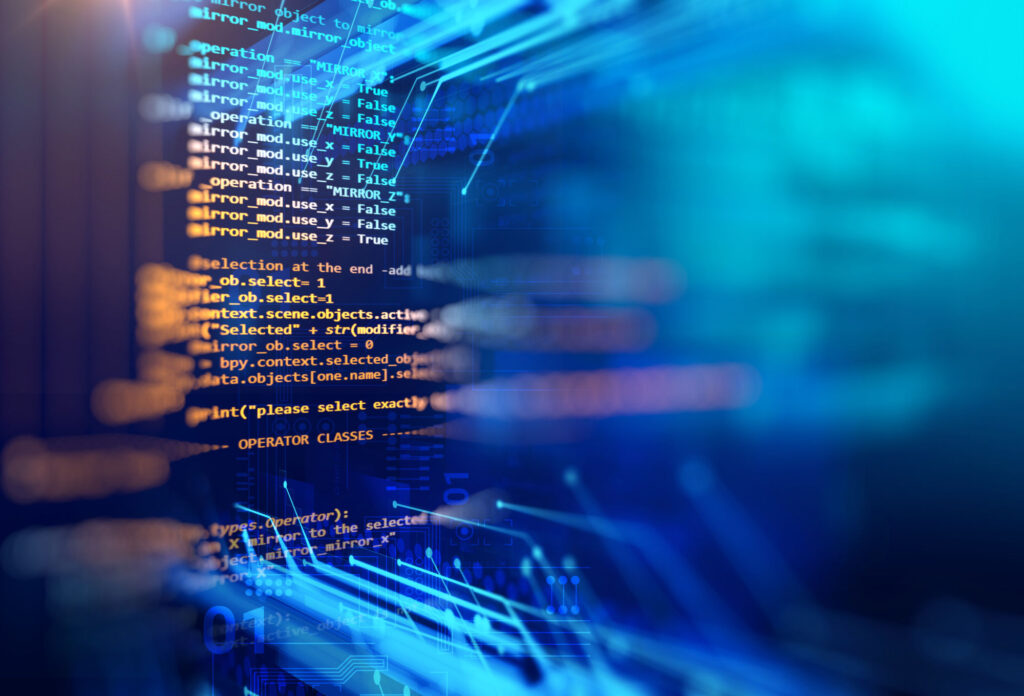
APEX is a Salesforce server-side programming language. It enables developers to build complex transaction logic, query data, modify objects and even call external REST APIs. APEX is a strongly typed language with Java-like classes and runtime, and deployment similar to source code compilation (i.e., an erroneous class cannot be deployed).
Sometimes however, there is a need to call APEX code dynamically at run-time.
For example, Nextian uses dynamic code tasks to support automation, integration & orchestration of service delivery. Users can write their own classes, deploy to their org and use in Nextian workflows by passing class and method names as configuration parameters without modifying the Nextian core package.
The required functionality written in pseudo-code looks as follows:
final String className ='MyHelperClass'; final String methodName = 'myHelperMethod'; Object result = call(className, methodName, args);
Important | The method signature (arguments and returned value) must be known to the caller. |
Salesforce provides a Callable interface to support dynamic calling of methods; however, it does not work for static methods.
This post demonstrates how to modify the Callable pattern to support static methods.
Modifying the Callable pattern for static methods
For the sake of discussion, let’s assume that two custom objects are available in Salesforce:
- Project__c — representing a project
- Task__c — tasks underneath the project
The objective is to enable tasks to call a static method with the assumption that the task object id is passed as an argument and there is no return value.
This can be achieved by modifying the standard Callable pattern as follows:
/** * Example helper class, this class must be deployed on the org (not anonymous APEX), * otherwise 'static can only be used on methods of a top level type' compilation error will occur. */ public class HelperClass implements Callable { /** * Dynamically called static method. */ public static void completeTask(Work_Order_Task__c t) { System.debug('Completing task ' + t.Id); } ... other methods ... /** * Callable::call() implementation, calling static function (completeTask, rather than this.completeTask) */ public Object call(String action, Map<String, Object> args) { switch on action { when 'completeTask' { // It is best to keep the naming consistent with the original method signature, error checking is // omitted: completeTask((Work_Order_Task__c)args.get('t')); // call() expects an object to be returned, null is returned for void function, other types can be used as needed: return null; } ... handle other methods ... when else { // A standard Salesforce exception is used for simplicity: throw new CalloutException('Method not implemented'); } } } }
The calling code would look as follows:
// Data for the dynamic call: final String className = 'HelperClass'; final String methodName = 'completeTask'; Work_Order_Task__c t = [SELECT Id, Name FROM Work_Order_Task__c LIMIT 1]; // Obtain callable interface (this instantiates the class, but since the class has only one-non static member there's no overhead): Callable c = (Callable) Type.forName(className).newInstance(); Object o = c.call( methodName, // objects new Map<String, Object> { // argument map 't' => t });
Conclusions
Dynamic APEX enables creation of highly flexible applications with configuration-driven run-time behavior.
Dynamic APEX can be extended to handle static methods creating a way to dynamically call helper classes. This enables advanced integration, automation and other scenarios without a need to modify pre-existing APEX code.
Nextian is a vendor of Quote-to-Cash (QTC) software for cloud and communications helping providers accelerate growth and increase customer lifetime value.
Contact us today to find out how we can help you!
Related posts
Salesforce data access tools overview
Discover options and tools for Salesforce data access, migration, integration, synchronization and reporting.
Best practices for managing account types in Salesforce
An overview of best practices for handling different account types (partners, resellers, vendors, customers) in Salesforce.
Integrating Salesforce with third-party billing systems for subscription services
A blueprint for integrating Salesforce with third party billing systems to create a seamless Quote-to-Cash process for subscription services.
GET THE NEXTIAN ADVANTAGE
We help enterprises increase revenue, profitability and gain efficiencies by realizing the full potential of the Salesforce platform.